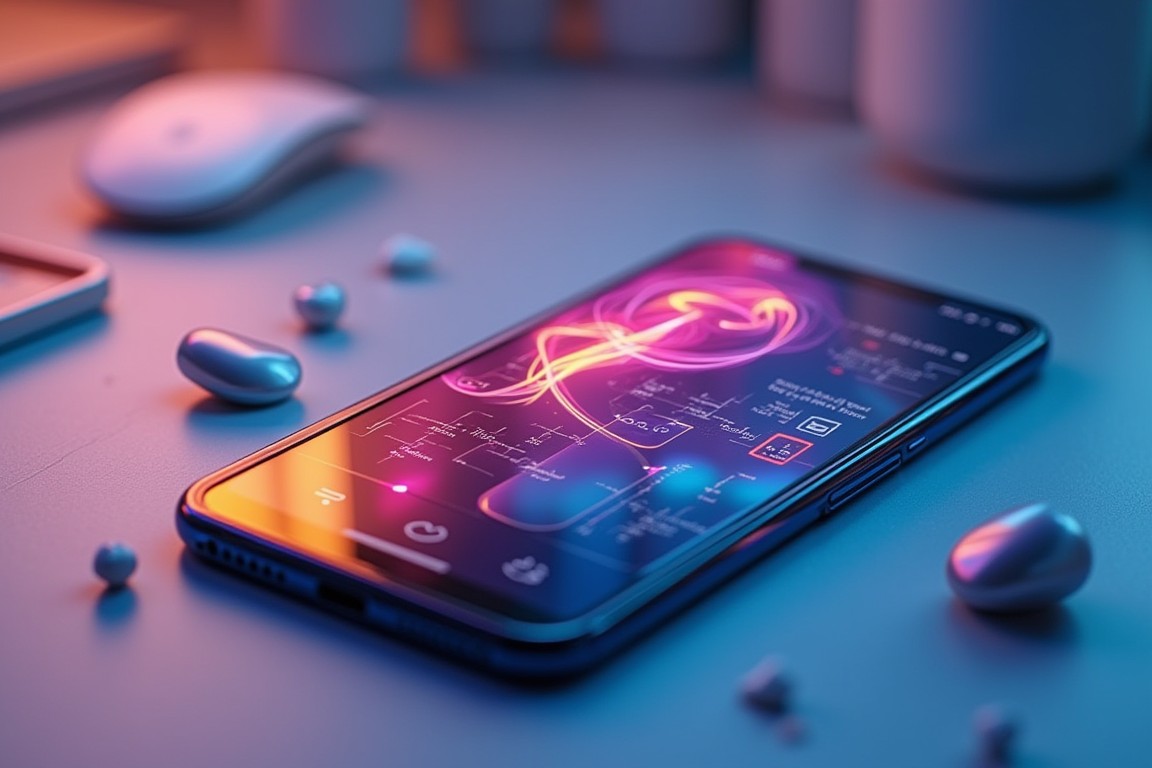
Introduction: Optimising React Native for Seamless User Experiences
React Native has become a popular framework for building cross-platform mobile applications, offering the promise of writing code once and deploying it on both iOS and Android. However, achieving optimal performance in React Native requires careful consideration and strategic implementation. Developers must navigate the complexities of bridging the JavaScript world with native components, ensuring a seamless user experience.
This article aims to provide actionable insights and practical tips to improve the performance of your React Native applications. By understanding and implementing these strategies, you can build apps that are not only functional but also fast and responsive. For complex projects, you may want to partner with a Mobile App Development Company that has expertise in React Native.
1. Optimise Images and Assets
Images and assets are often the largest contributors to app size and can significantly impact performance. Optimising them is crucial for a smooth user experience.
Use WebP Format
WebP is a modern image format that provides superior compression compared to JPEG and PNG. Using WebP can significantly reduce image file sizes without sacrificing quality.
Resize Images Appropriately
Ensure that images are resized to the dimensions they are displayed in the app. Avoid using large images that are scaled down, as this wastes memory and processing power.
Utilise Asset Bundling
Bundle your assets to reduce the number of network requests. This can improve loading times, especially on slower networks.
Lazy Load Images
Implement lazy loading for images that are not immediately visible. This defers the loading of images until they are needed, improving initial loading times.
2. Minimise Bridge Crossings
React Native uses a bridge to communicate between the JavaScript thread and the native thread. Minimising bridge crossings can significantly improve performance.
Batch State Updates
Batch state updates to reduce the number of bridge crossings. This means updating multiple state variables at once, rather than individually.
Use Native Modules
For performance-critical operations, consider using native modules. Native modules allow you to write code in native languages like Java or Objective-C, bypassing the bridge.
Avoid Excessive Logging
Excessive logging can slow down your app. Disable logging in production builds to improve performance.
Use shouldComponentUpdate
or React.memo
Implement shouldComponentUpdate
or React.memo
to prevent unnecessary re-renders. This can significantly reduce the number of bridge crossings.
3. Optimise Lists and Scroll Views
Lists and scroll views are common components in mobile apps. Optimising them is essential for smooth scrolling and rendering.
Use FlatList
or SectionList
Use FlatList
or SectionList
for rendering large lists. These components are optimised for performance and provide features like virtualisation.
Implement getItemLayout
Implement getItemLayout
for FlatList
or SectionList
. This allows the component to calculate the layout of items without rendering them, improving performance.
Virtualise Long Lists
Virtualise long lists to render only the items that are visible on the screen. This reduces memory usage and improves rendering performance.
Avoid Inline Styles
Avoid using inline styles in list items. Inline styles can cause unnecessary re-renders.
4. Manage State Effectively
Effective state management is crucial for building performant React Native apps.
Use useState
and useReducer
Wisely
Use useState
and useReducer
hooks wisely. Avoid storing unnecessary data in the state, as this can lead to unnecessary re-renders.
Implement Memoisation
Implement memoisation using useMemo
and useCallback
hooks. This can prevent unnecessary calculations and re-renders.
Use Context API Sparingly
Use the Context API sparingly. The Context API can cause unnecessary re-renders if used improperly.
Consider Third-Party State Management Libraries
For complex apps, consider using third-party state management libraries like Redux or MobX. These libraries provide a more structured approach to state management.
5. Optimise JavaScript Code
Optimising your JavaScript code can significantly improve the performance of your React Native app.
Avoid Expensive Calculations
Avoid performing expensive calculations in the render method. This can slow down rendering and lead to janky animations.
Use requestAnimationFrame
Use requestAnimationFrame
for animations and UI updates. This ensures that animations are smooth and synchronised with the device’s refresh rate.
Minify JavaScript Code
Minify your JavaScript code in production builds. This reduces the file size and improves loading times.
Profile Your Code
Profile your code to identify performance bottlenecks. Use tools like the React Native Profiler to identify slow components and functions.
6. Utilise Native Modules for Performance-Critical Operations
For performance-intensive tasks, consider using native modules. Native modules allow you to write code in native languages like Java or Objective-C, bypassing the JavaScript bridge.
Implement Native Modules
Implement native modules for tasks that require high performance, such as image processing or complex calculations.
Use Native Libraries
Use native libraries for tasks that are already implemented in native code. This can save development time and improve performance.
Bridge Communication
Use the bridge communication efficiently. Avoid sending large amounts of data across the bridge.
Test Native Modules Thoroughly
Test your native modules thoroughly to ensure they are working correctly and not causing any performance issues.
7. Profile and Debug Performance Issues
Profiling and debugging performance issues is essential for identifying and resolving bottlenecks.
Use React Native Profiler
Use the React Native Profiler to identify slow components and functions. This tool provides insights into the performance of your app.
Use Device Profiling Tools
Use device profiling tools like Xcode Instruments or Android Profiler to identify performance issues at the native level.
Use Debugging Tools
Use debugging tools like the React Native Debugger to identify and fix bugs.
Conduct Performance Testing
Conduct performance testing on different devices and network conditions. This helps you identify and address performance issues in various scenarios.
Table: Performance Optimisation Tips
Tip | Description | Benefit |
---|---|---|
Optimise Images | Use WebP, resize images | Reduced app size |
Minimise Bridge Crossings | Batch updates, native modules | Improved performance |
Optimise Lists | Use FlatList , virtualise lists |
Smooth scrolling |
Manage State Effectively | useState , memoisation |
Reduced re-renders |
Optimise JavaScript | Avoid expensive calculations | Faster rendering |
Utilise Native Modules | Implement native modules | High performance |
Profile and Debug | Use profiler, debugging tools | Identify bottlenecks |
Keynotes:
- Optimise images and assets for reduced app size.
- Minimise bridge crossings for improved performance.
- Optimise lists and scroll views for smooth scrolling.
- Manage state effectively to reduce re-renders.
- Optimise JavaScript code for faster rendering.
- Utilise native modules for performance-critical operations.
- Profile and debug performance issues to identify bottlenecks.
FAQ (Frequently Asked Questions):
1. Why is optimising images important in React Native?
Optimising images reduces app size and improves loading times, leading to a better user experience.
2. What are bridge crossings in React Native, and why should they be minimised?
Bridge crossings are the communication between the JavaScript and native threads. Minimising them improves performance by reducing overhead.
3. How can I optimise lists and scroll views in React Native?
Use FlatList or SectionList, implement getItemLayout, virtualise long lists, and avoid inline styles.
4. What are native modules, and when should I use them?
Native modules allow you to write code in native languages, bypassing the JavaScript bridge. Use them for performance-critical tasks.
5. What tools can I use to profile and debug performance issues in React Native?
Use the React Native Profiler, device profiling tools like Xcode Instruments or Android Profiler, and debugging tools like the React Native Debugger.
Achieving Peak Performance in React Native
Optimising a React Native application for peak performance is a continuous process that requires attention to detail and a deep understanding of the framework’s architecture. By implementing the seven tips outlined in this article, developers can significantly enhance the user experience, ensuring smooth animations, fast loading times, and efficient resource utilisation. Remember, each application has unique performance requirements, and it is crucial to profile and test thoroughly to identify specific bottlenecks. Whether you’re working independently or as part of a Mobile App Development team, a commitment to performance optimisation will ultimately lead to more successful and user-friendly mobile applications.